UserNotifications
Quick Start
Scheduling local notifications
Scheduling local notifications
"NotificationsTesting" Would Like to Send You Notifications
Notifications may include alerts, sounds, and icon badges. These can be configured in Settings.
--------------------------
| Dont't Allow | Allow |
--------------------------
Asking permission to use notifications
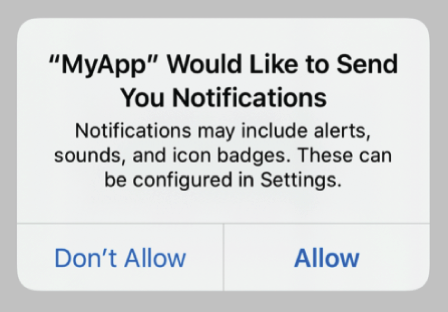
The ability to post noninterrupting notifications provisionally to the Notification Center.
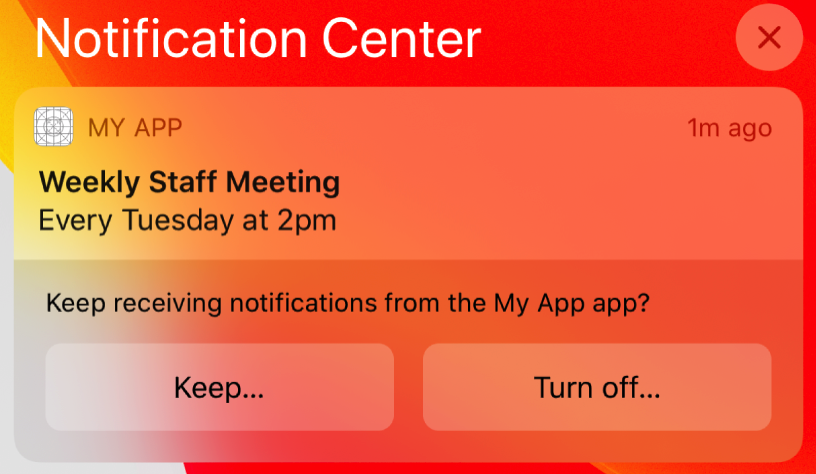
let center = UNUserNotificationCenter.current()
center.requestAuthorization(options: [.alert, .sound, .badge, .provisional]) { granted, error in
if let error = error {
// Handle the error here.
}
// Provisional authorization granted.
}
Unlike explicitly requesting authorization, this code doesn’t prompt the user for permission to receive notifications. Instead, the first time you call this method, it automatically grants authorization. However, until the user either explicitly keeps or turns off the notification, the authorization status is UNAuthorizationStatus.provisional. Because users can change the authorization status at any point, you should still check the status before scheduling local notifications.
Authorization Status
Customize notifications based on the current authorizations
let center = UNUserNotificationCenter.current()
center.getNotificationSettings { settings in
guard (settings.authorizationStatus == .authorized) ||
(settings.authorizationStatus == .provisional) else { return }
if settings.alertSetting == .enabled {
// Schedule an alert-only notification.
} else {
// Schedule a notification with a badge and sound.
}
}
The above example uses a guard condition to prevent the scheduling of notifications if the app isn’t authorized. The code then configures the notification based on the types of interactions allowed, preferring the use of an alert-based notification whenever possible.
Full Code
import SwiftUI
import UserNotifications
import AlertToast
struct ContentView: View {
@State private var showToast = false
@State private var alertTitle = ""
var body: some View {
VStack {
Image(systemName: "globe")
.imageScale(.large)
.foregroundColor(.accentColor)
Text("Hello, world!")
Button("Request Permission") {
// First, Authorization
UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .badge, .sound]) { success, error in
if success {
showToast.toggle()
alertTitle = "Success"
} else if let error = error {
print(error.localizedDescription)
}
}
}
.alert(alertTitle, isPresented: $showToast) {}
Button("Schedule Notification") {
// Second, Schedule Notification
let content = UNMutableNotificationContent()
content.title = "Feed the cat"
content.subtitle = "It looks hungry"
content.sound = UNNotificationSound.default
// show this notification five seconds from now
let trigger = UNTimeIntervalNotificationTrigger(timeInterval: 5, repeats: false)
// choose a random identifier
let request = UNNotificationRequest(identifier: UUID().uuidString, content: content, trigger: trigger)
// add our notification request
UNUserNotificationCenter.current().add(request)
showToast.toggle()
alertTitle = "Notification is coming"
}
}
.padding()
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Open System Settings
System Notification Authorization Status
#pragma mark - Notifications
/// 添加系统通知状态的观察者
- (void)addObserverForSystemNotificationStatus {
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(checkNotificationAuthorization)
name:UIApplicationWillEnterForegroundNotification
object:nil];
}
// 在 checkNotificationAuthorization 方法中检查通知权限
- (void)checkNotificationAuthorization {
[[UNUserNotificationCenter currentNotificationCenter] getNotificationSettingsWithCompletionHandler:^(UNNotificationSettings * _Nonnull settings) {
dispatch_async(dispatch_get_main_queue(), ^{
BOOL newAuthorizationStatus = self.isSystemNotificationOn;
if (settings.authorizationStatus == UNAuthorizationStatusAuthorized) {
newAuthorizationStatus = YES;
} else {
newAuthorizationStatus = NO;
}
// iOS 12.0 新增了 Provisional 的状态,无需获得用户授权也可发送 Deliver Quietly 的通知
if (@available(iOS 12.0, *)) {
if (settings.authorizationStatus == UNAuthorizationStatusProvisional) {
newAuthorizationStatus = YES;
}
}
// 刷新表格
if (newAuthorizationStatus != self.isSystemNotificationOn) {
self.isSystemNotificationOn = newAuthorizationStatus;
[self.tableView reloadData];
}
});
}];
}
Open App Settings From System Settings
providesAppNotificationSettings
- (void)userNotificationCenter:(UNUserNotificationCenter *)center openSettingsForNotification:(UNNotification *)notification {
NSURL *url = [NSURL URLWithString:@"https://google.com"];
[self openTrdPartyURL:url];
}
iOS 12.0+
An option indicating the system should display a button for in-app notification settings.
Preparing Your App For iOS 12 Notifications
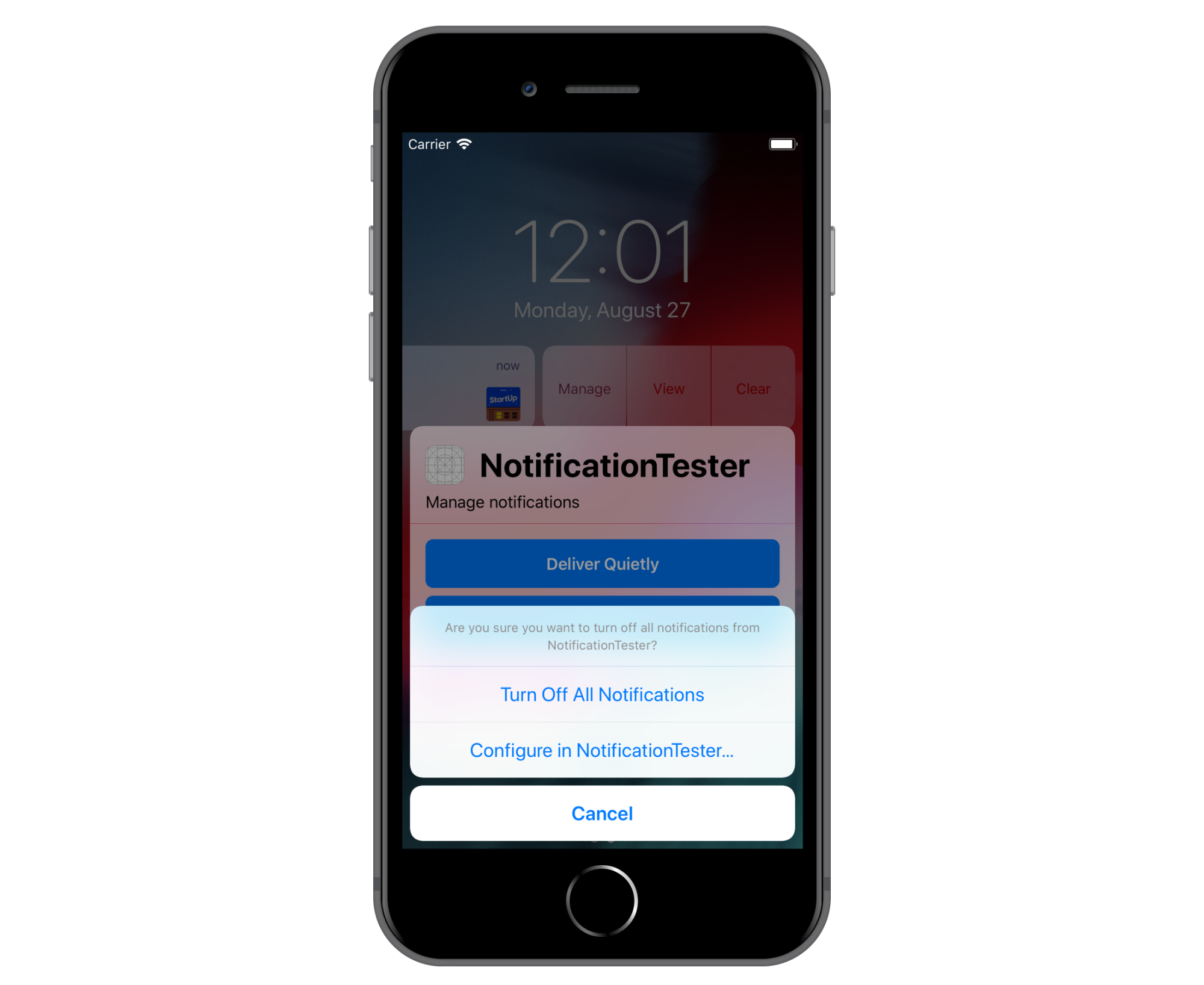
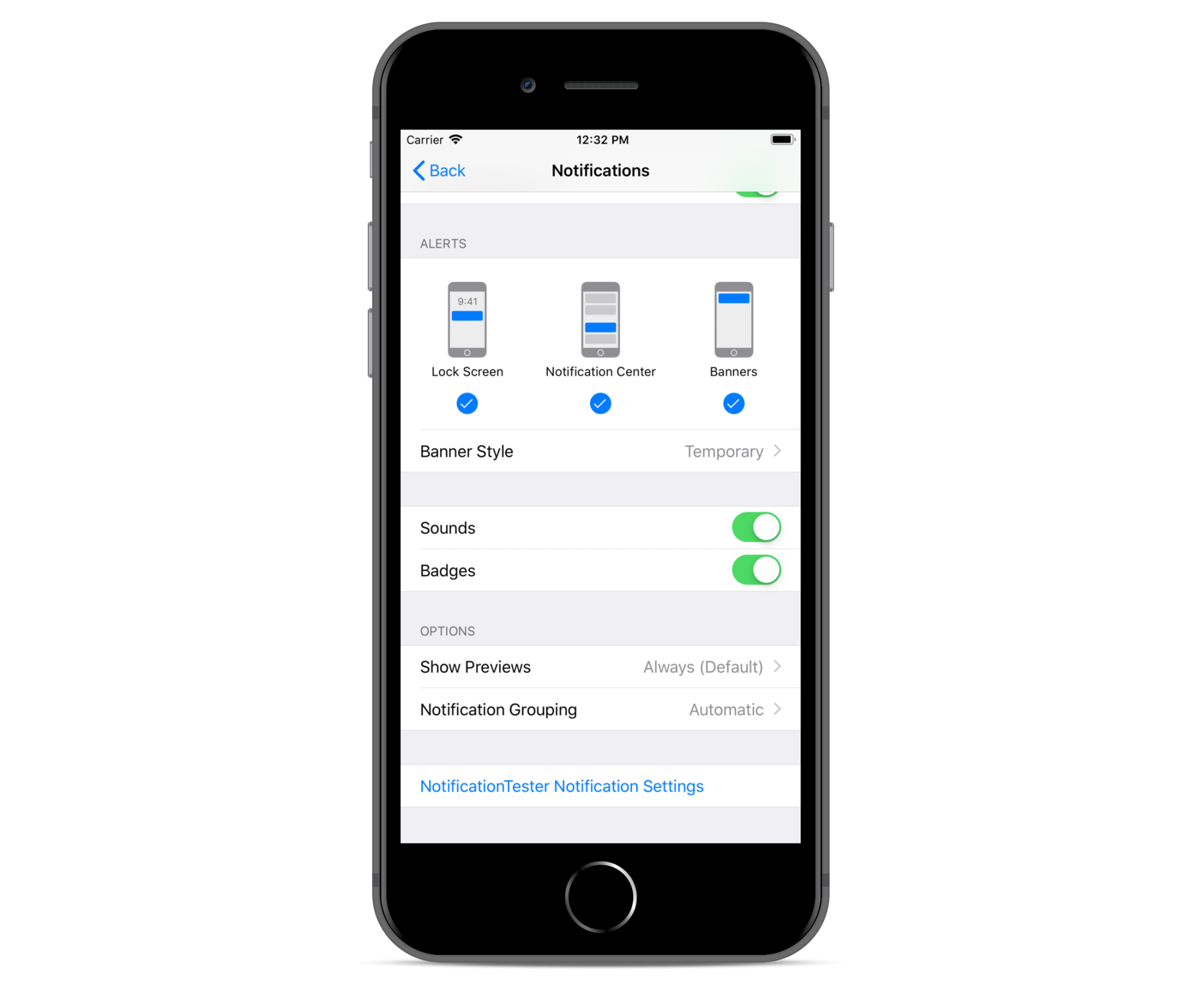